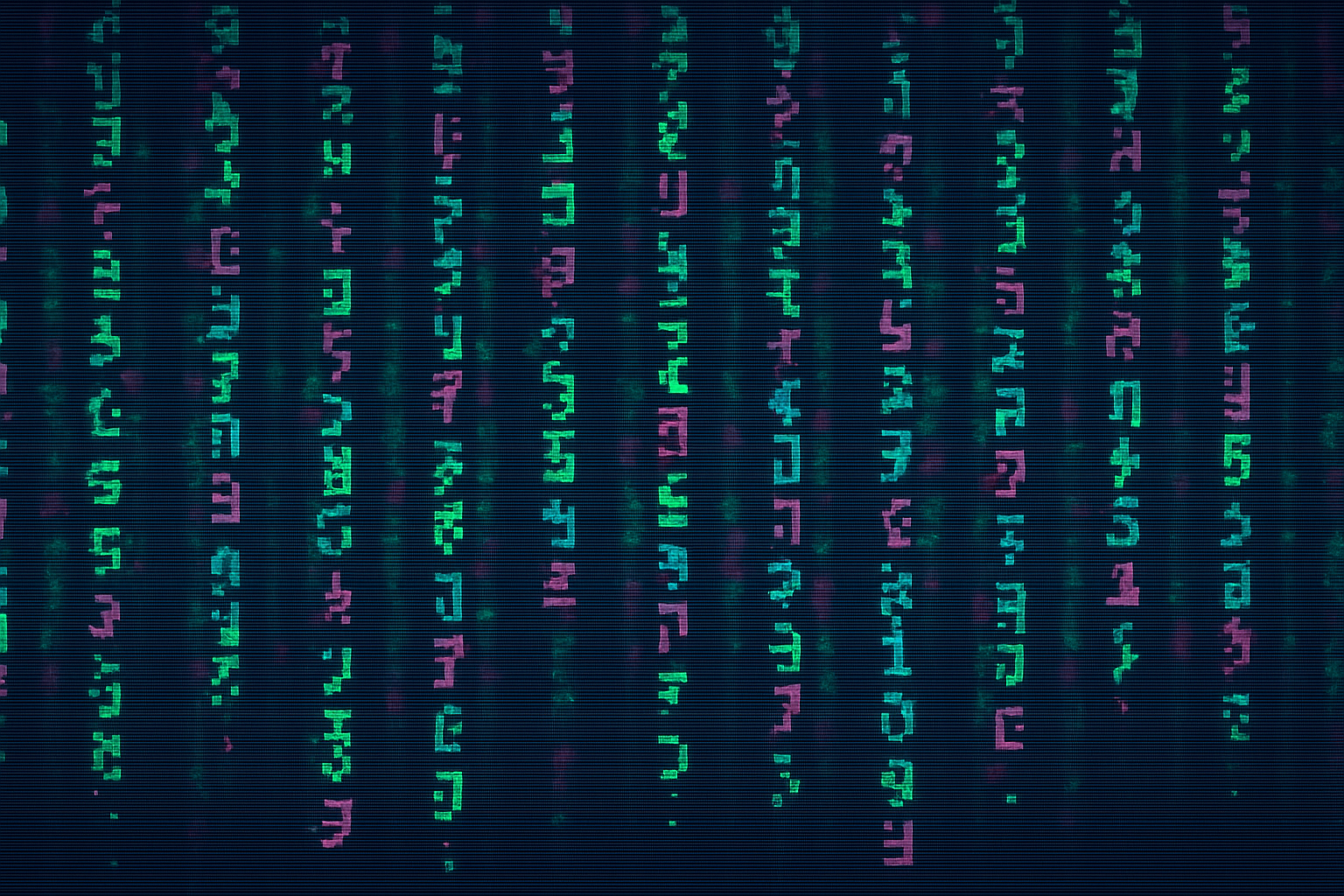
Encode the Matrix: Browser Text Effects for Fun and No Profit
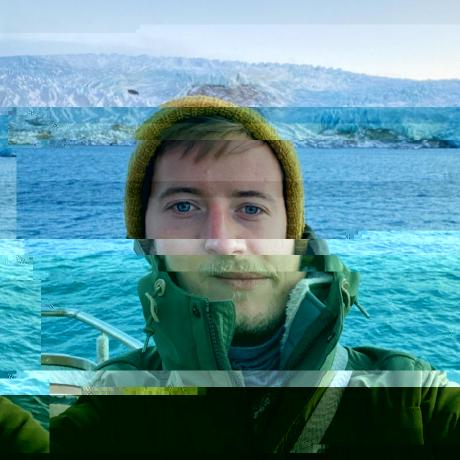
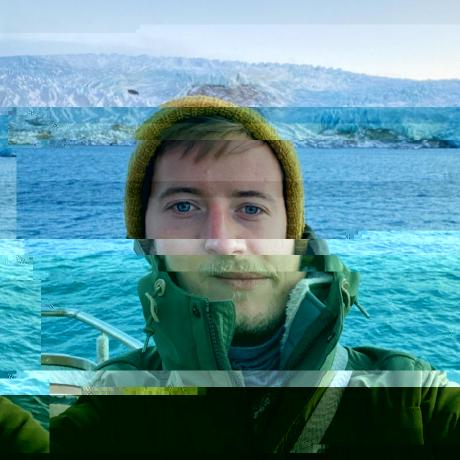
Sometimes, you want to build something for fun, but it turns out to be pretty useful. I've always wanted to build something like the Matrix Effect. There are plenty of examples out there already, but it felt like something a cyberpunk-obsessed '90s kid should build.
An early prototype of how I wanted to approach the effect ended up a little different, but it turns out to be useful for all sorts of applications I never would have considered.
From interactive game interfaces to web applications, being able to easily apply transformations and animations to arbitrary strings is pretty great.
I wrapped the code up into a text transformation Component for React and open-sourced the code.
It's as easy to use as:
npm add @interwebalchemy/ciph3r-text
Note: You can use bun
, yarn
, pnpm
, etc., too. JavaScript Registry (jsr
) support is coming soon.
import Ciph3rText from "@interwebalchemy/ciph3r-text";
export default function Component() {
return <Ciph3rText defaultText="Hello, world!" action="decode" />;
}
Note: This is currently React-only because that's what I was working on at the time, but if there's interest, I can port it to other frameworks or release a framework-agnostic build via Mitosis or vanilla JavaScript.
By default, Ciph3rText
uses a pretty standard set of alphanumeric characters and basic symbols, but unicode can get pretty wild. Like, positively Lovecraftian.
For the tech demo I created for the Winter 2025 Recurse Center Game Jam - that was the first video above - I had a lot of fun gathering unique characters to use with Ciph3rText
.
Here are some examples of just a few of the unique Unicode characters you can use:
- Anglo-Saxon Runes:
ᚳ᛫ᛗᚨᚷᛚᛋᛖᚩᛏᚪᚾᛞᚻᛁᚱᚧ᛬ᚠᛇᛒᛦᚦᚢᚹᚳᚫ
- Old Irish Runes:
᚛ᚉᚑᚅᚔᚋᚈᚍᚂᚐᚌᚓ᚜
- Math Symbols:
⏀⏁⏂⏃⏄⏅⏆⏇⏈⏉⏊⏋⏌⏍
- Miscellaneous Symbols:
çリクƵツᐊ╌¿£⭔
- Blocks:
▒ ░ █ ▚ ▞ ▜ ▝
The <Ciph3rText />
component also has a number of configurable properties to control the effect's behavior:
defaultText
(required): the text to display during server rendering, after decoding, or before encoding or transformingaction
: controls whether the text transformation usesencode
,decode
,transform
, orscramble
logic; defaults toencode
targetText
*: the text to transform into when usingaction="transform"
onFinish
**: callback to execute when thedefaultText
has been fullydecode
d,encode
d, ortransform
editerationSpeed
: how frequently the logic to change characters executes;120
forencode
/decode
/scramble
and150
fortransform
maxIterations
**: how many times the logic to change characters can run;36
forencode
/decode
and54
fortransform
characters
: a limited string of characters that you want to use in the effect; defaults to [alphanumeric English characters and basic symbols](view source)additionalCharacters
: an optional string of characters that you want to use in addition to thecharacters
; this provides an easy way to have a base character set and add specific characters for different effects
* required if action="transform"
** not applicable if action="scramble"
Usage Tips:
- For more interesting effects, like the ones in the Lost Signal demo, you may want to break your string into chunks of random sizes and apply varying text colors,
iterationSpeed
s, etc., to each chunk. - Find some unique characters to use. Maybe get inspired by the Matrix or add some cursed diacritics?
- You can leverage the
onFinish
callback to swapdefaultText
andtargetText
or change theaction
to create effects chains. - You can use the
characters
andaddtionalCharacters
properties to dynamically change the characters used in the effect.